7 min read
— views•Get Started with Playdate SDK on MacOS
This post is quite old and no longer being updated. The following information may no longer work the with the latest version of the tools, libraries, frameworks, or best-practices discussed.
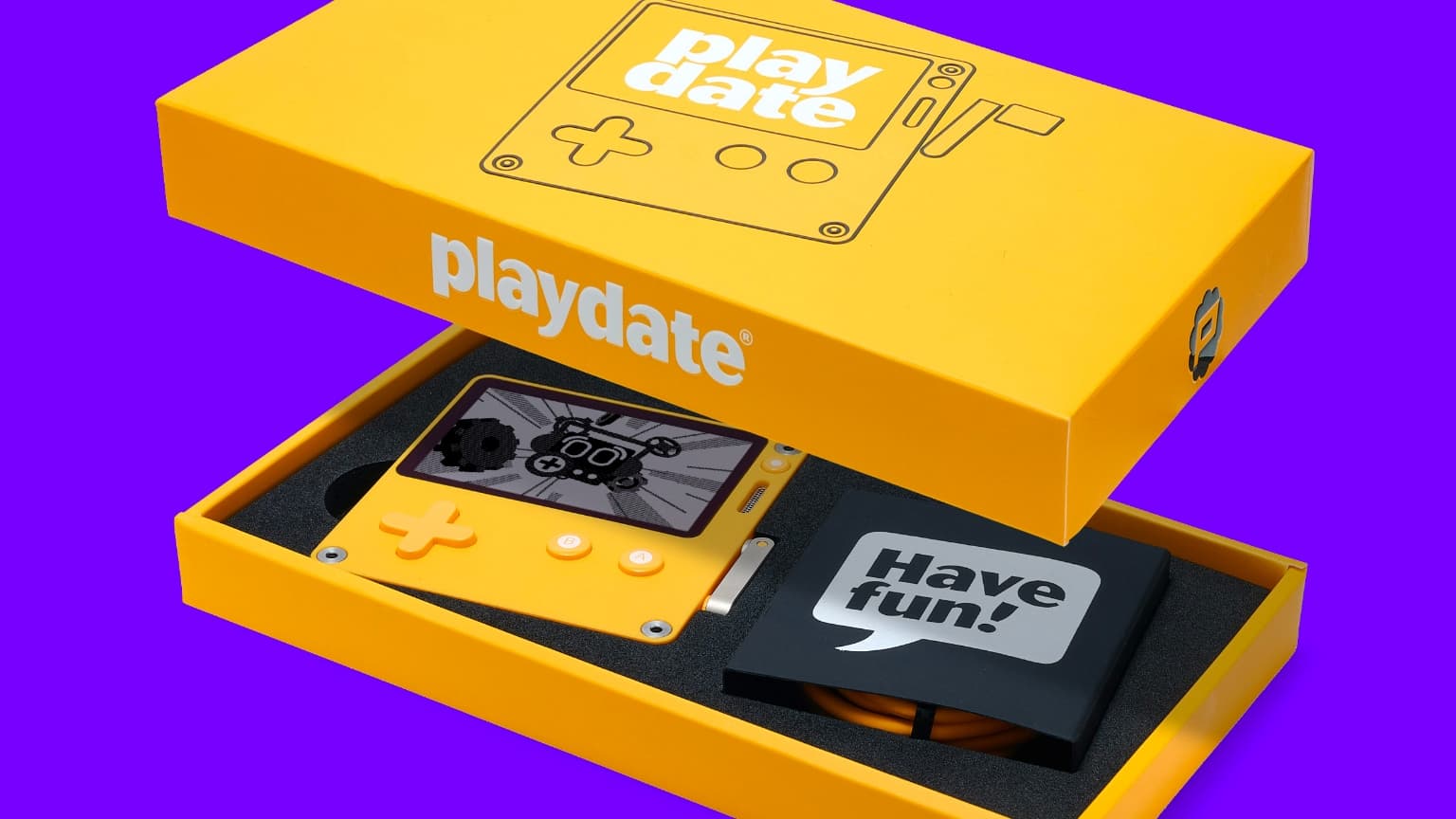
Playdate is an upcoming handheld gaming device produced by Panic and designed in collaboration with Teenage Engineering.
In this guide, I'll show you how to get started developing your own Playdate game using VSCode on MacOS. If you're familiar with basic game development concepts and comfortable writing code in C or Lua, skip the primer and disclaimer and jump ahead to the good stuff. Otherwise, read on.
#IntroductionThere are two things that are incredibly exciting to me about the upcoming Playdate device. First, I have an affinity for 1bit pixel art, and second, every Playdate works as a development kit out of the box.
Game development and programming in general can feel inaccessible to non software engineers. Panic has set out to create something that while incredibly niche, offers a low barrier to entry to get starting making your own game for the device.
There are two distinct ways of approaching game development on the Playdate; Playdate Pulp and Playdate SDK. Pulp is a browser based game creation tool that requires little to no programming knowledge. With Pulp, you can create simple games and learn about the fundamentals of game development if you are coming in completely fresh to the world of programming. The SDK is a traditional game development environment, you'll use an IDE to code your game, compile the code to a game file, and sideload the game onto a Playdate simulator. The SDK allows you to write your game using either C or Lua programming languages.
In this guide, I will show you how to get going using the SDK and Lua. Lua is a lightweight, high-level programming language with a C API that compiles down to bytecode. If you're proficient in C then go ahead and use that instead.
If you're brand new and curious about trying Pulp, I recommend checking out SquidGodDev on YouTube.
#Design ConsiderationsBefore getting started, it is worth noting that the playdate resolution is 400x240px
and the display is 2.7in
. The pixel density is fairly high for such a small screen. Because of this, traditional sprite and image sizes may appear small. A GameBoy-style 16x16
character sprite would need to be doubled in size (32x32
) to appear the same size on the Playdate. Ultimately, this is a stylistic choice, but it is worth noting before you spend hours laboring over hand-crafted 1bit pixel art (don't ask me how I know 😒).
With that primer out of the way, let's get started configuring the development environment! You will be expected to know the basics of game development and it is up to you to write testable Lua code toward the end steps of this guide. If you're just getting started with Lua and game development, but you have familiarity with other programming languages, you should be good to go. Just refer to the documentation (more on that below). If this sounds good, continue reading.
#Download Playdate SDKOpen the Playdate Dev page and follow the instructions to download the SDK. Open the .zip you previously downloaded and double-click the PlaydateSDK.pkg
file. Step through the installation and install to the default Developer
directory in your home folder.
The Playdate API docs are included locally in the root of the PlaydateSDK
folder. If you're using Lua, you will want to use Inside Playdate.html
.
brew install lua
bashIf you don't have homebrew installed, check out their official installation instructions.
Install the following VSCode plugin: Playdate by Orta. Once the extension is installed, while in VSCode, press f1 or shift+command+p
. Search > settings.json
and select Preferences: Open Settings (JSON). Add the following code to your settings.json
file:
{ "Lua.diagnostics.globals": ["playdate", "import"], "Lua.workspace.library": [ "/Users/yourHomeFolderName/Developer/PlaydateSDK/CoreLibs" ],}
jsonMake sure to update yourHomeFoldername
to your home folder. To find your home folder, open Finder and open your home folder using shift+command+h
.
Inside the Developer
directory where you installed the PlaydateSDK
, create a new folder for your game (name anything you'd like): YourGameName
. Open YourGameName
in VSCode and create a new directory called .vscode
.
Create two new files inside of the .vscode
directory: launch.json
and tasks.json
.
Paste the following inside launch.json
and update YourGameName
to include the game name you chose for the game directory earlier.
// launch.json{ "version": "0.2.0", "configurations": [ { "request": "launch", "type": "playdate", "name": "Run app in Playdate simulator", "preLaunchTask": "Compile App", "source": "${workspaceFolder}/Source", "output": "${workspaceFolder}/../PlaydateSDK/Disk/Games/YourGameName.pdx", } ]}
jsonNow open tasks.json
and paste the following, edit YourGameName
:
// tasks.json{ "version": "2.0.0", "tasks": [{ "label": "Compile App", "command": "pdc", "args": ["Source", "../PlaydateSDK/Disk/Games/YourGameName.pdx"], "type": "shell" }]}
jsonIn the root directory of your game project, create three folders: Source
, images
, and sounds
.
In the Source
, make a new file called lua.main
. This is the first file that is loaded by the Playdate.
-- lua.main
import "CoreLibs/object"
import "CoreLibs/graphics"
import "CoreLibs/sprites"
local function initalize()
--your code here
end
initalize()
function playdate.update()
--your code here
end
luaBy this point you are ready to start adding your own code. All that is left now is to test the configuration of our Playdate extension and project file structure.
If you have zero clue what do at this point but are curious to see if everything is set up properly, that is okay! Try just loading a simple background image into the simulator. Create an 400x200px image called background.png
, put it in your project's images
folder, then add the following to lua.main
:
-- lua.main
import "CoreLibs/graphics"
import "CoreLibs/sprites"
local gfx <const> = playdate.graphics
local function initalize()
playerImage = gfx.image.new("images/player")
local backgroundImage = gfx.image.new("images/background")
gfx.sprite.setBackgroundDrawingCallback(
function(x, y, width, height)
gfx.setClipRect(x, y, width, height)
backgroundImage:draw(0, 0)
gfx.clearClipRect()
end
)
end
initalize()
function playdate.update()
gfx.sprite.update()
end
luaNow the moment you've been working toward, with your initial game code in place, press f5
and your .pdx
compiled game will automatically compile and the Playdate Simulator will open with your game loaded.
If it doesn't work, there is likely an issue with your directory structure or task.json
/ launch.json
files. Double check you have these configured properly with your project directory name in place of the placeholders I provided.
If you plan to upload your project to GitHub or other repository, it's good practice to create a .gitignore
and README.md
file.
Since we're developing on Mac, you can start with the following. Add anything else as needed:
// .gitignore
.DS_Store
jsonCreate README.md
and add any relevant info about your project:
// README.md
# YourGameName
A Playdate game by YourName
...
markdownGood luck, and happy hacking! If you make something cool, or have any questions, hit me up on Twitter.
Big thanks to Orta for the excellent VSCode extension.
Enjoy this post? Like and share!